aaronfrey.com
A modern Django-based reboot of my Ruby on Rails blog platform built in 2012. Now with best practices and clean architecture. Features include content management, responsive design, and performance optimizations.
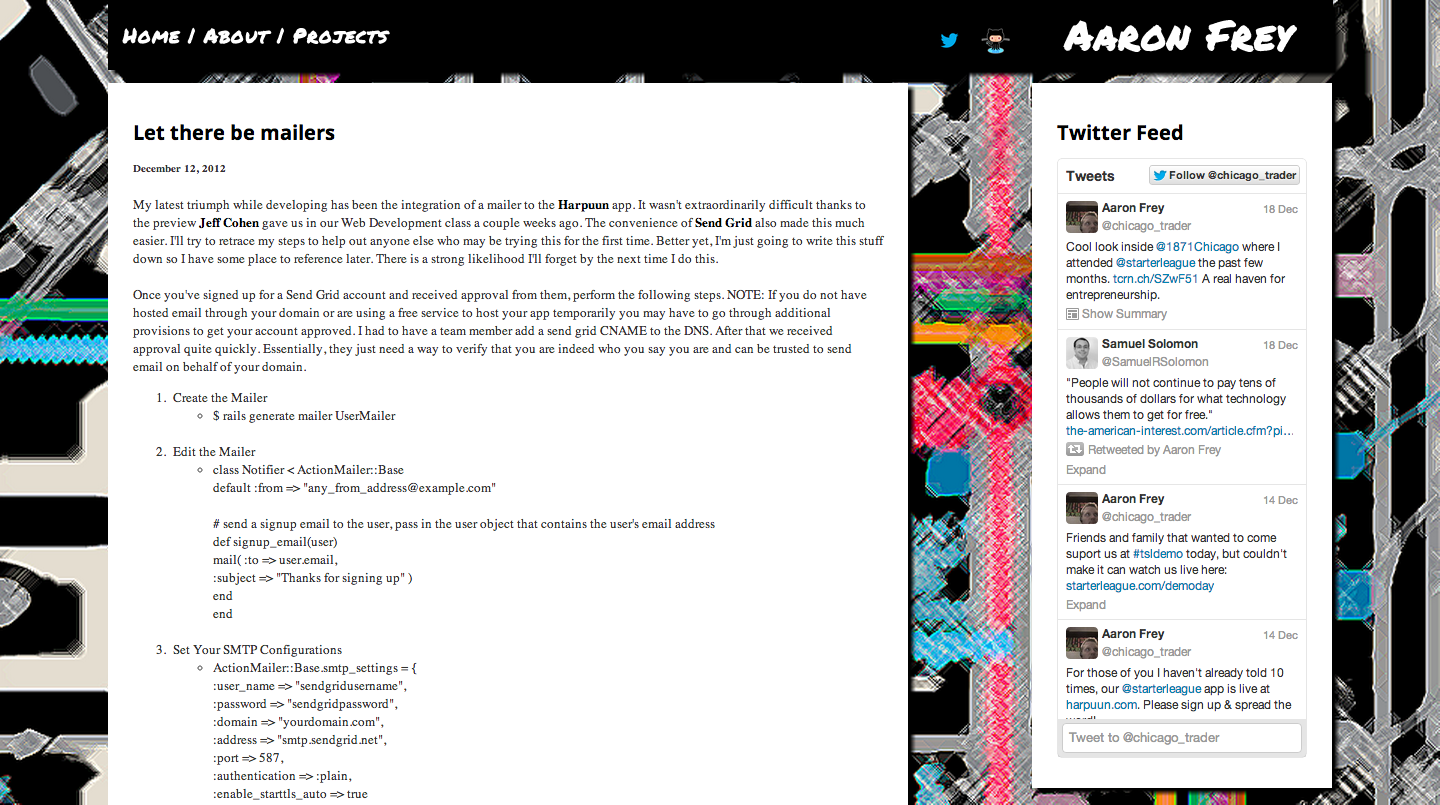
Key Features
- Content management system for creating, editing, and deleting blog posts
- Responsive design with a clean, user-friendly interface
- User authentication and authorization for secure post management
- PostgreSQL database for reliable data storage
- Dockerized development and deployment environment
Technical Highlights
- Django: Core framework for backend logic and admin interface
- PostgreSQL: Robust relational database for storing blog data
- Docker: Containerized environment for consistent development and deployment
- Whitenoise: Efficient static file serving for production
- Modular Design: Clear separation of concerns with apps for posts, users, and templates
Skills Demonstrated
- Backend Development: Django, PostgreSQL, RESTful API design
- Frontend Development: HTML, CSS, JavaScript, responsive design
- DevOps: Docker, containerization, and deployment best practices
- Testing: Unit tests, integration tests, and test-driven development
- Modern Python Practices: Type hints, modular code, and clean architecture
Future Improvements
- User Comments: Add a commenting system for blog posts
- Search Functionality: Implement full-text search for posts
- API Endpoints: Expose RESTful APIs for external integrations
- Advanced Caching: Add Redis for improved performance